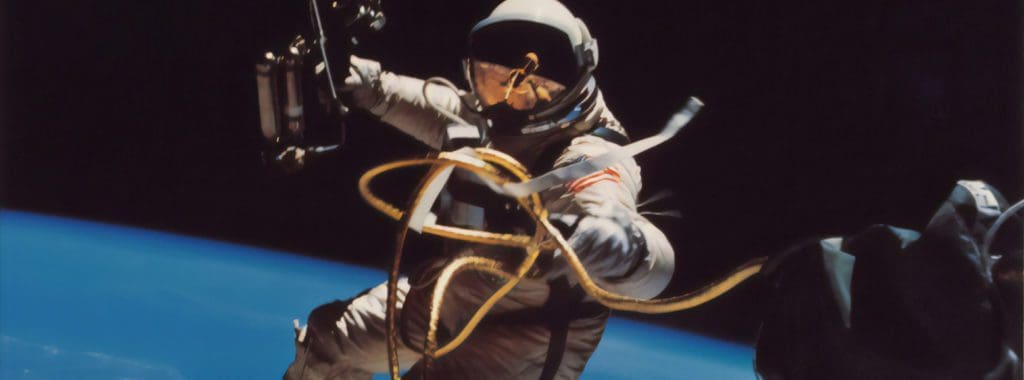
I want a div wrapping an image to be a specific height and width, I don’t want to skew the image and I want the image to be centered. I have run into this problem many times building websites over the years. I solved this annoying little issue today using jQuery!
The Simple Idea:
- Give a height, width and overflow hidden to your wrapper div
- On your image set a height of 100%, a width of auto and a display of inline-block
- With jQuery, find the width of the image. Subtract the width of the wrapper to find the total extra width. Then divide by 2 to get the number you want on each side. Finally add that number as a negative margin-left.
I hope this solves some little annoyances for you. Please reach out to us in the comments or through our contact page if you need any help with this or any other WordPress related issues you are having.
Here is an example:
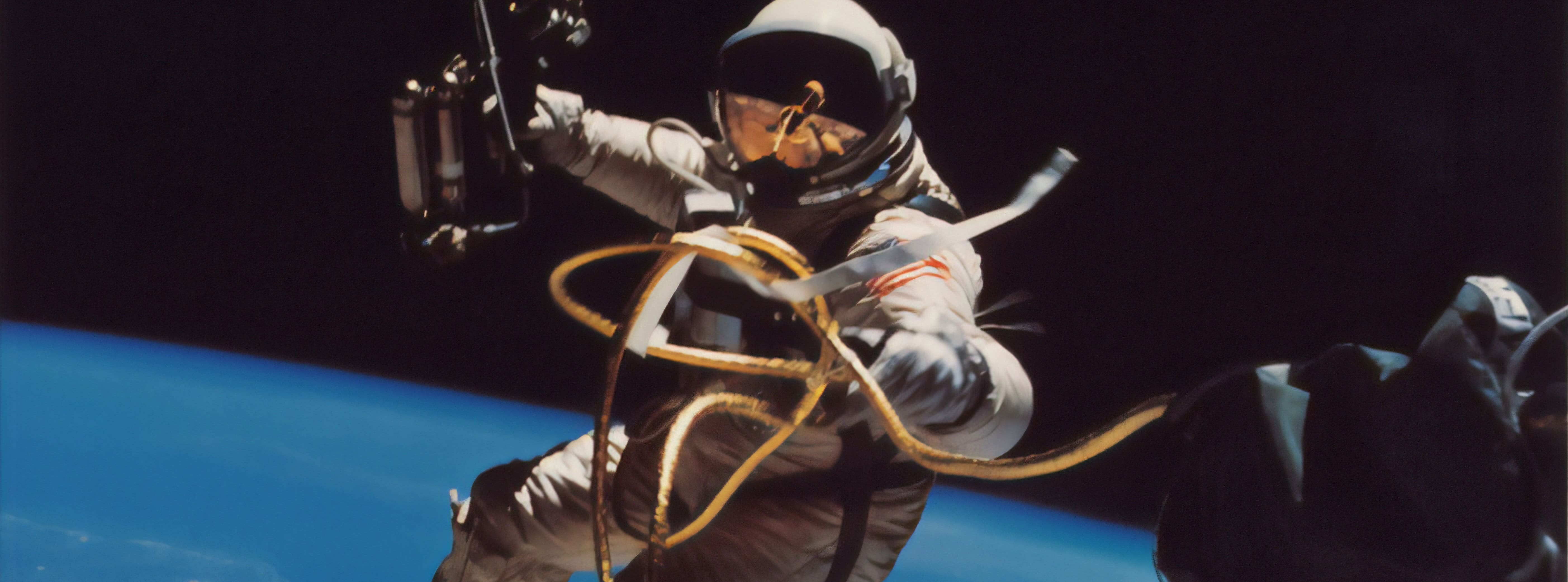
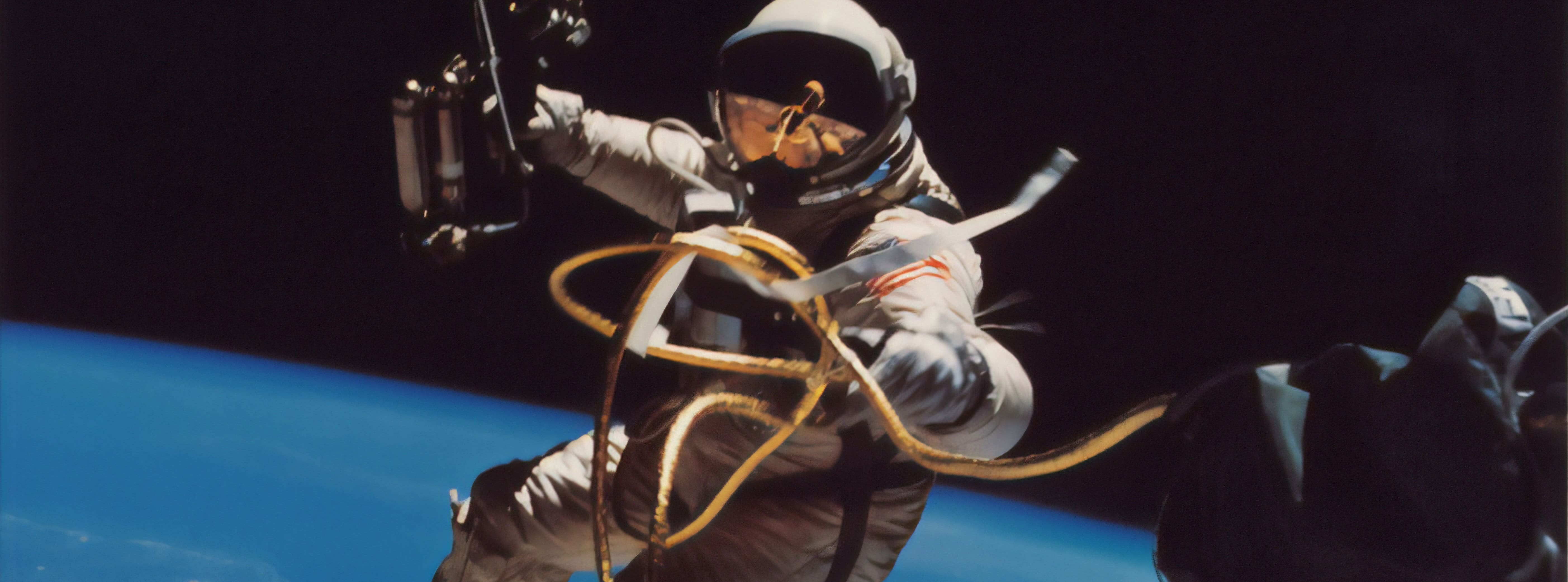
Here is the code:
HTML
<div class="image-wrapper">
<img src="/wp-content/uploads/2020/10/spaceman.jpg">
</div>
CSS
.image-wrapper {
height: 250px;
width: 300px;
overflow: hidden;
}
.image-wrapper img {
height: 100%;
width: auto;
display: inline-block
}
jQuery
jQuery(function($){ // Establishing the $
$(window).bind("load", function() { // Waiting until everything has loaded so your css hits first
$('.image-wrapper').each(function(){ // Find all image wrappers you want to effect
var wrapperwidth = $(this).width(); // Find the width of the image wrapper
var width = $(this).find('img').width();// Find the width of the image
var widthmargin = (width-wrapperwidth)/2; //Subtract the width by the wrapper width and divide by 2
$(this).find('img').css('margin-left', - widthmargin + 'px'); //add the margin as a negative margin-left
});
});
});